Contenidos
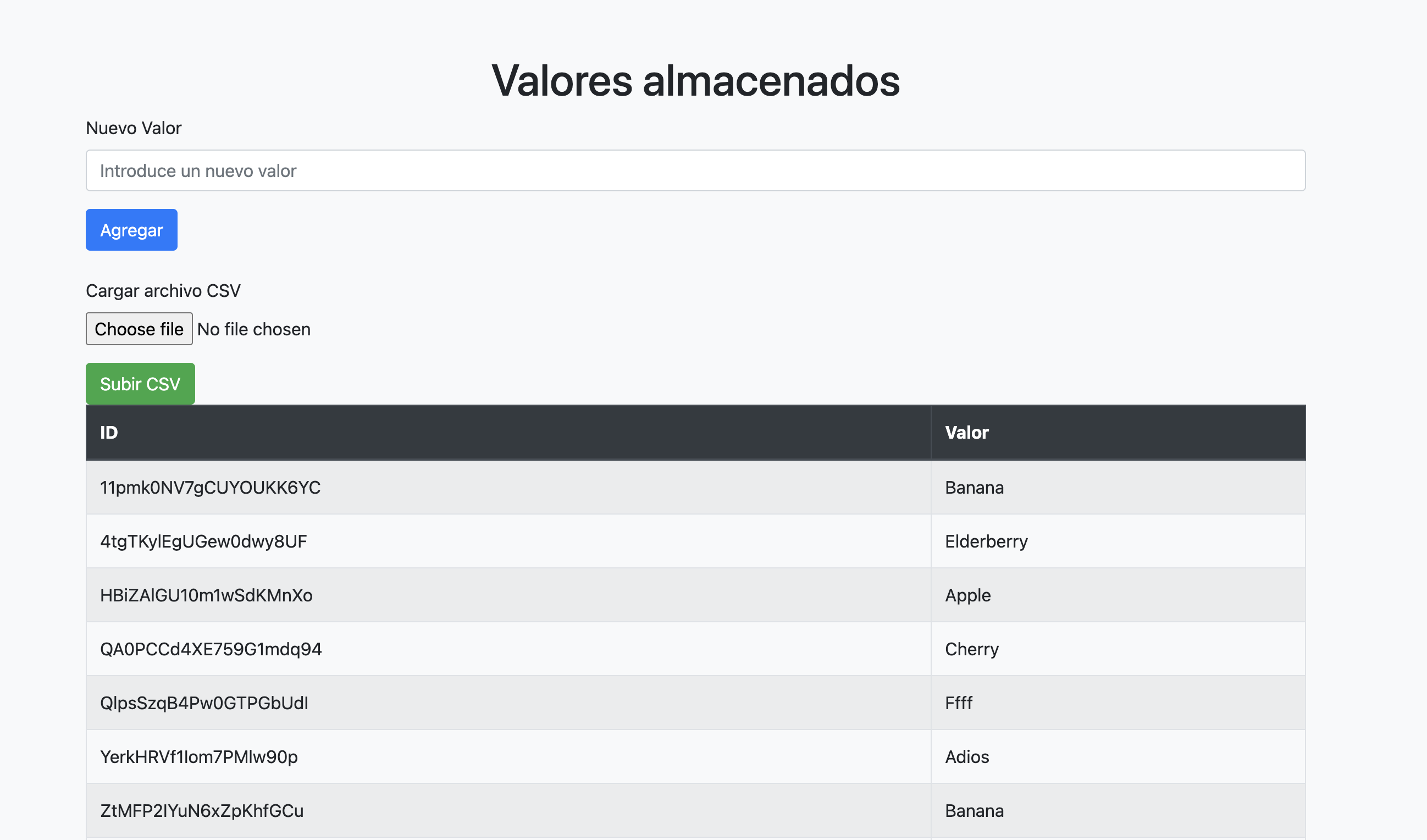
Estructura de la aplicación
1 2 3 4 5 6 7 |
importdata/ ├── api/ │ ├── templates/ │ │ └── values.html │ └── index.py └── key/ └── key.json |
Configurar Firebase
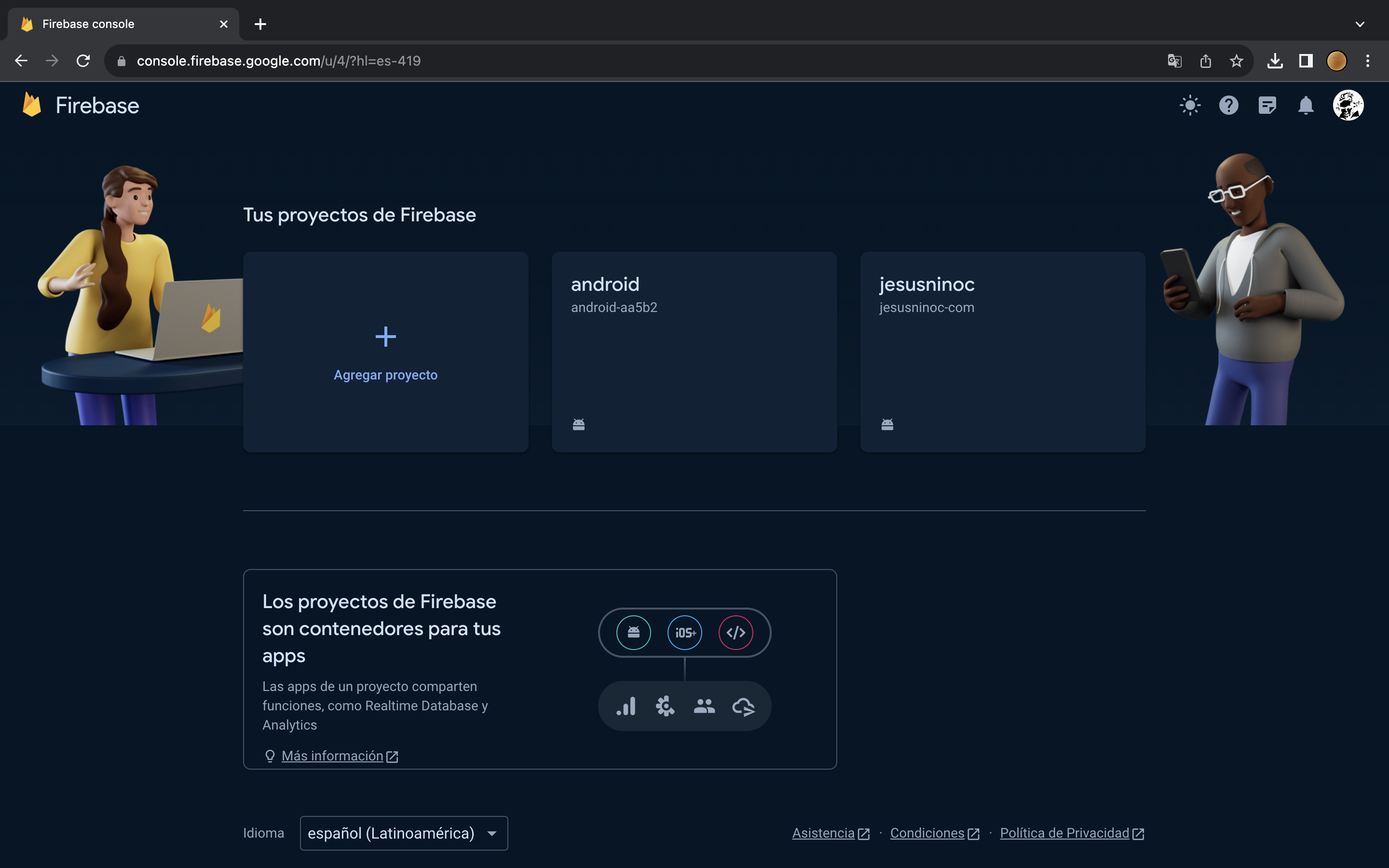
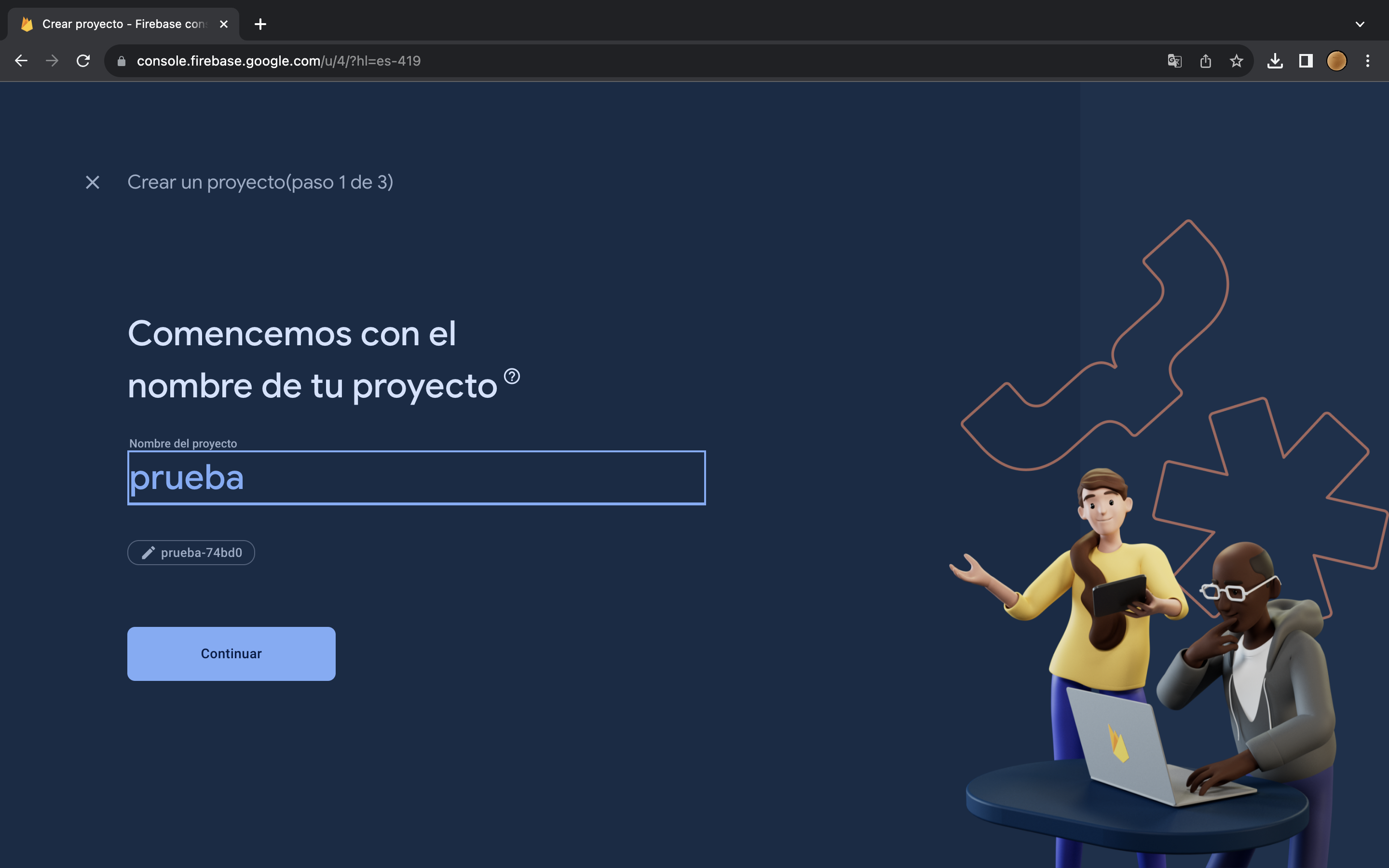
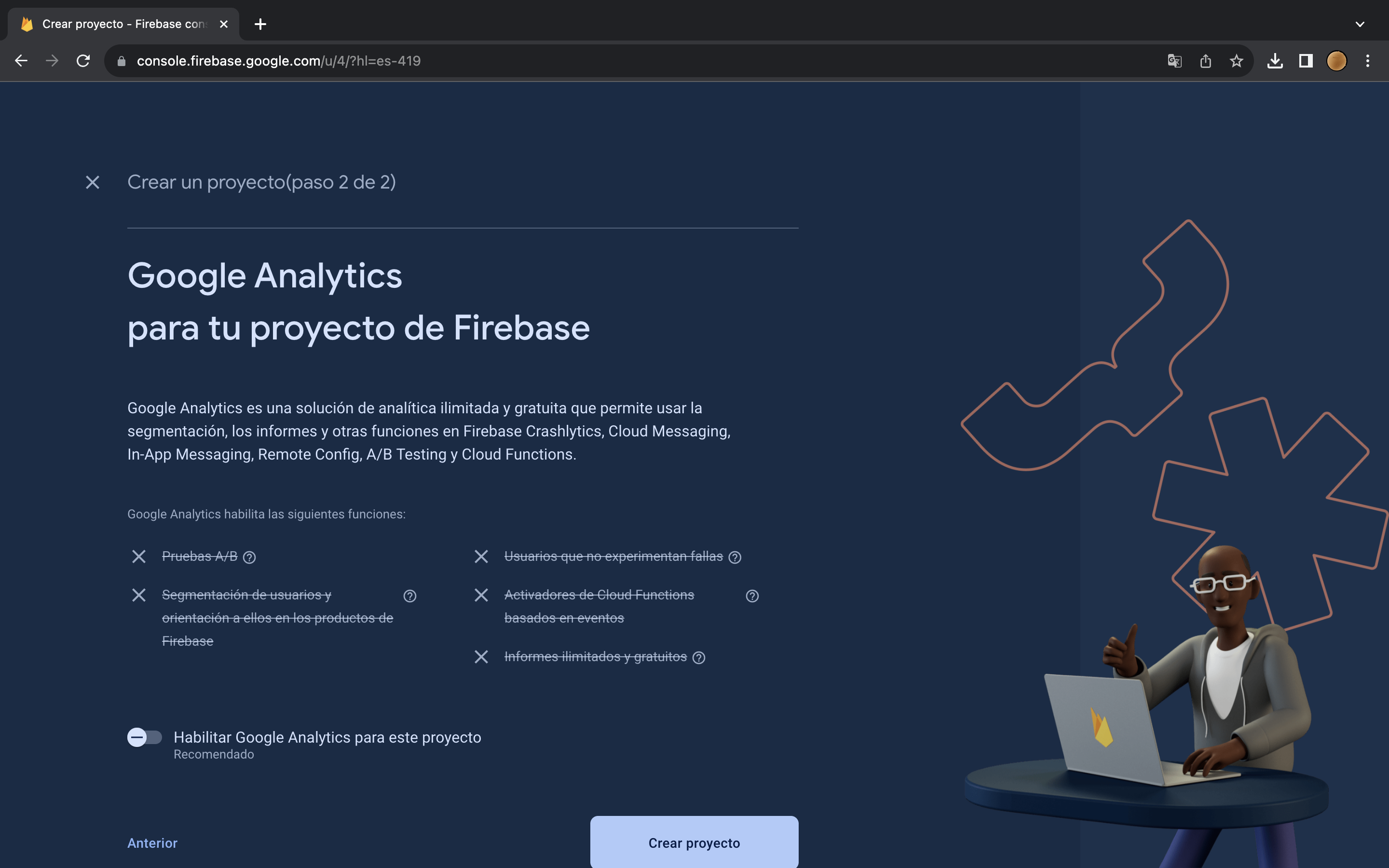
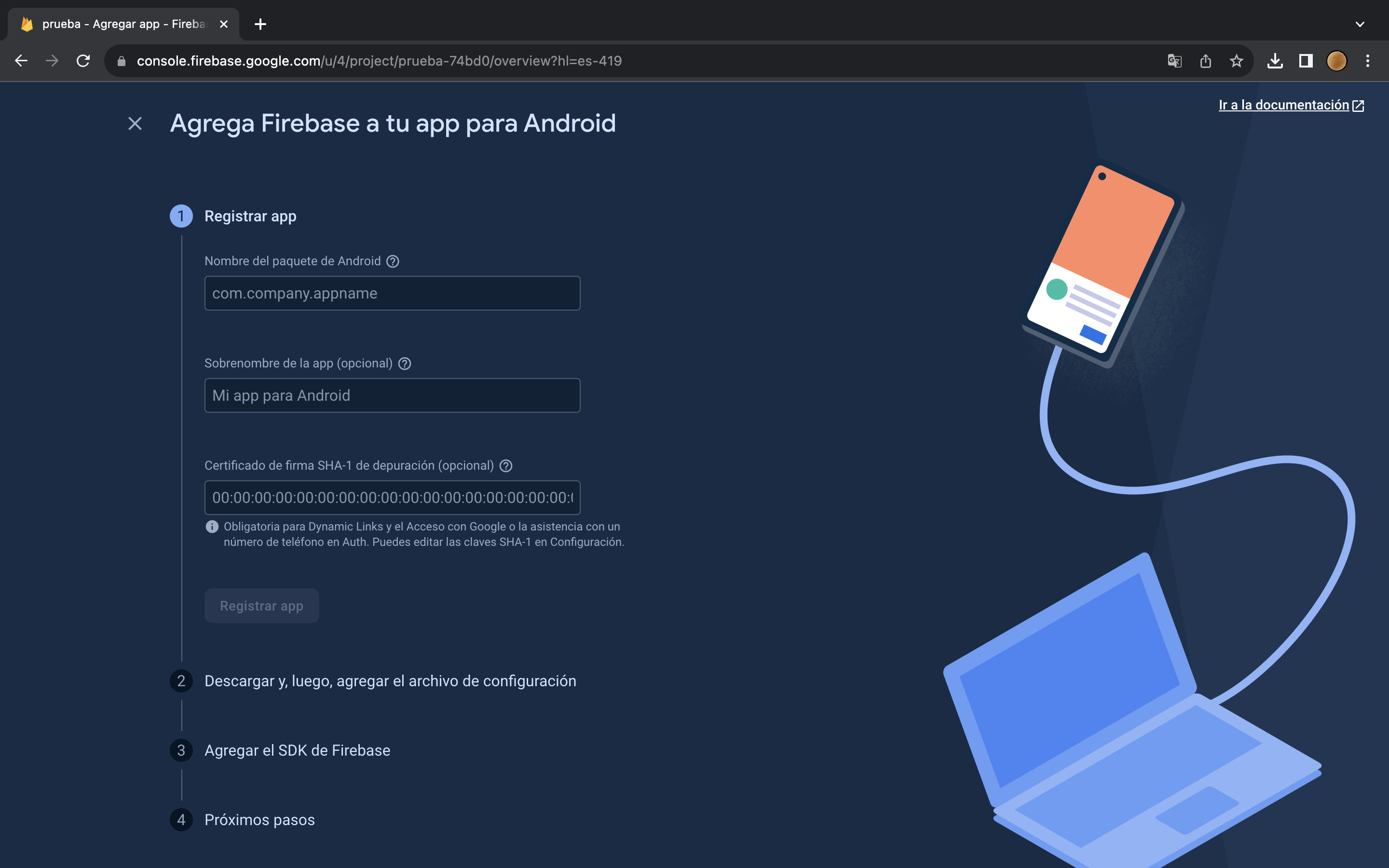
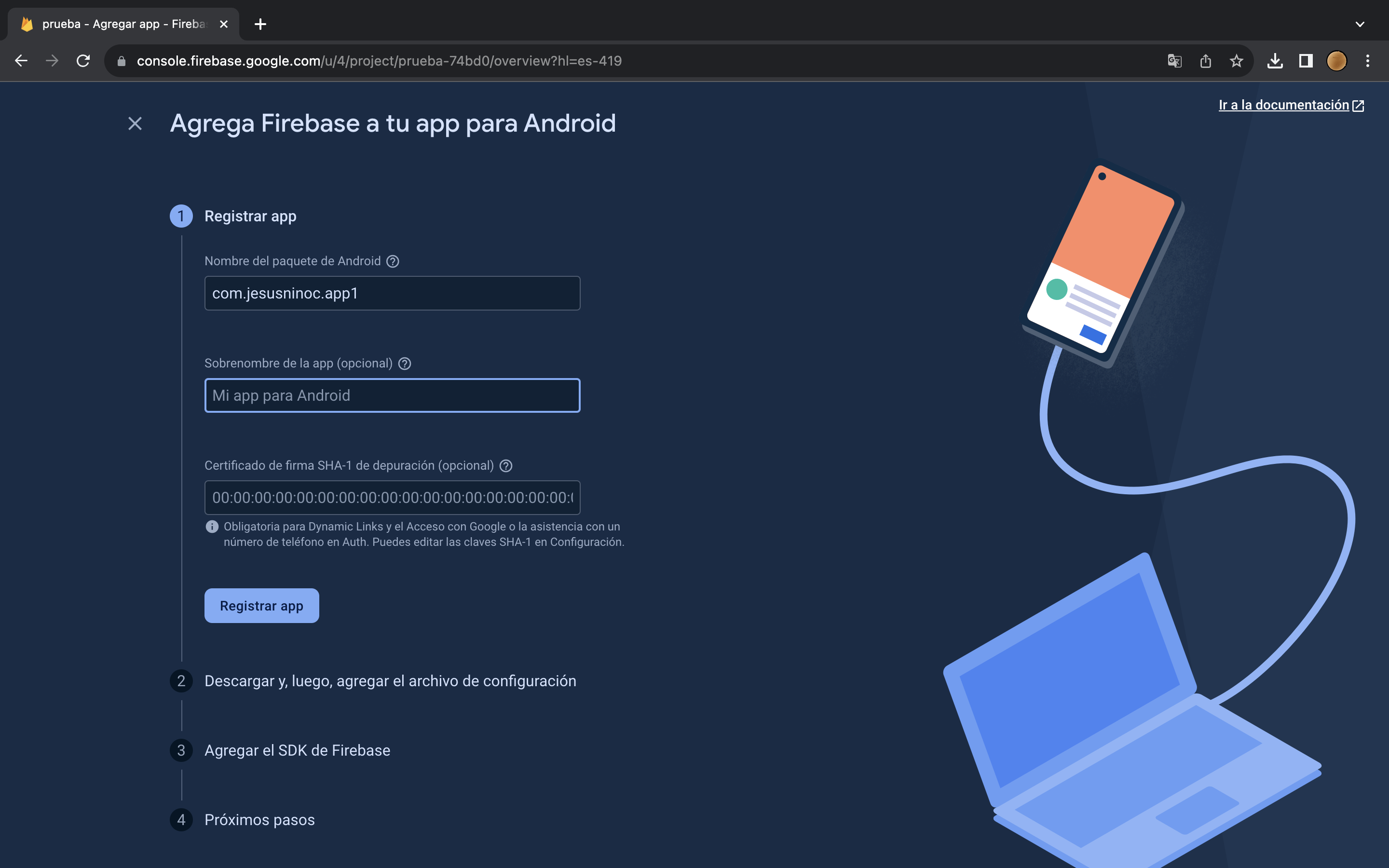
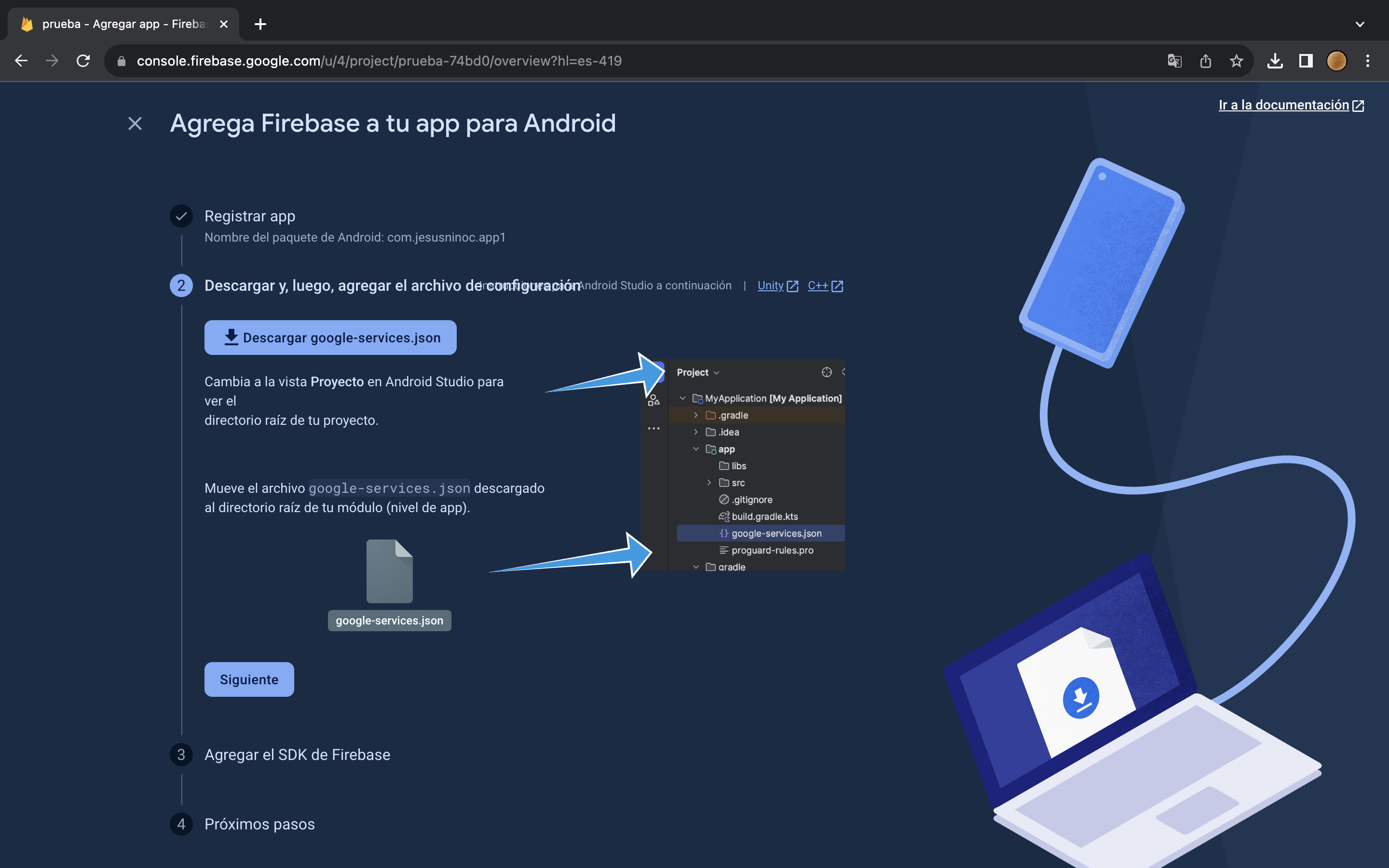
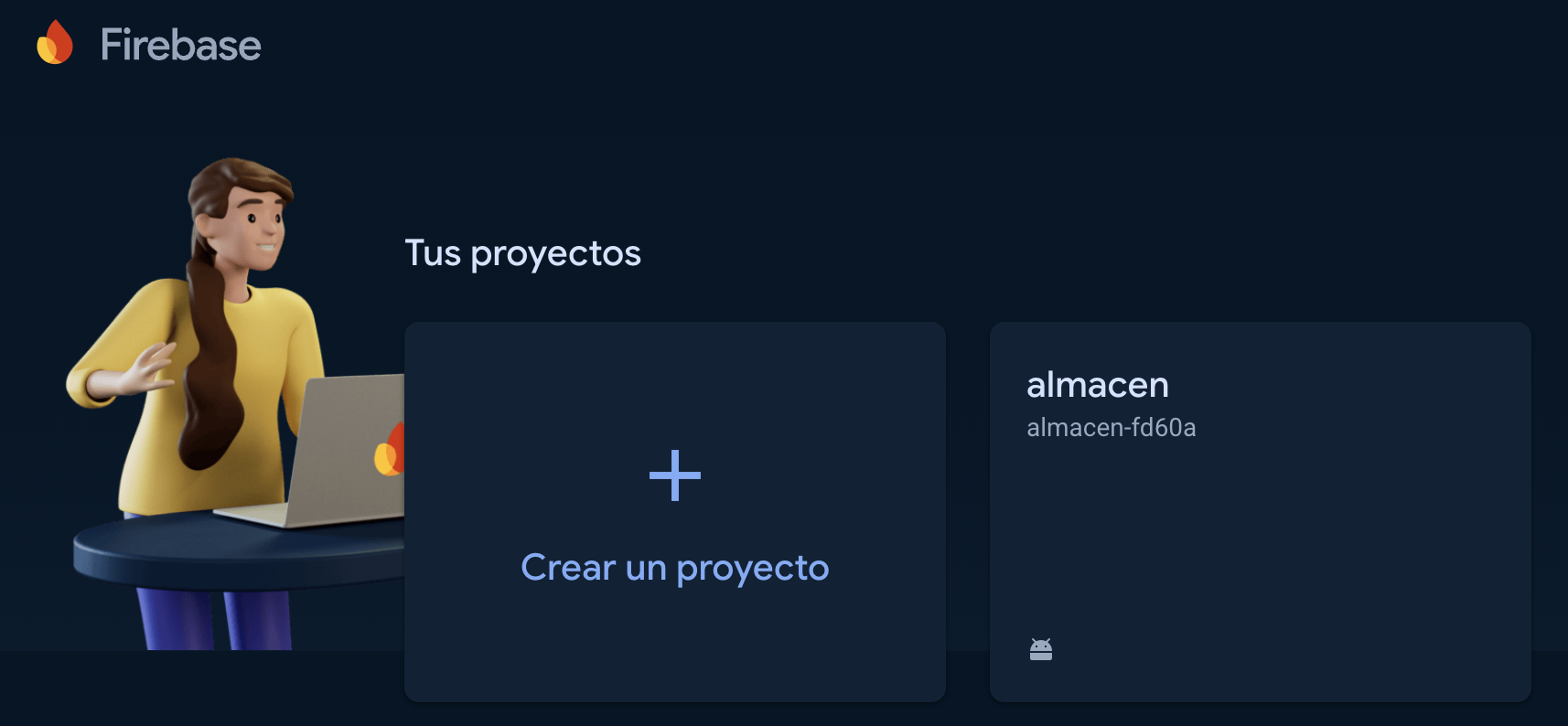
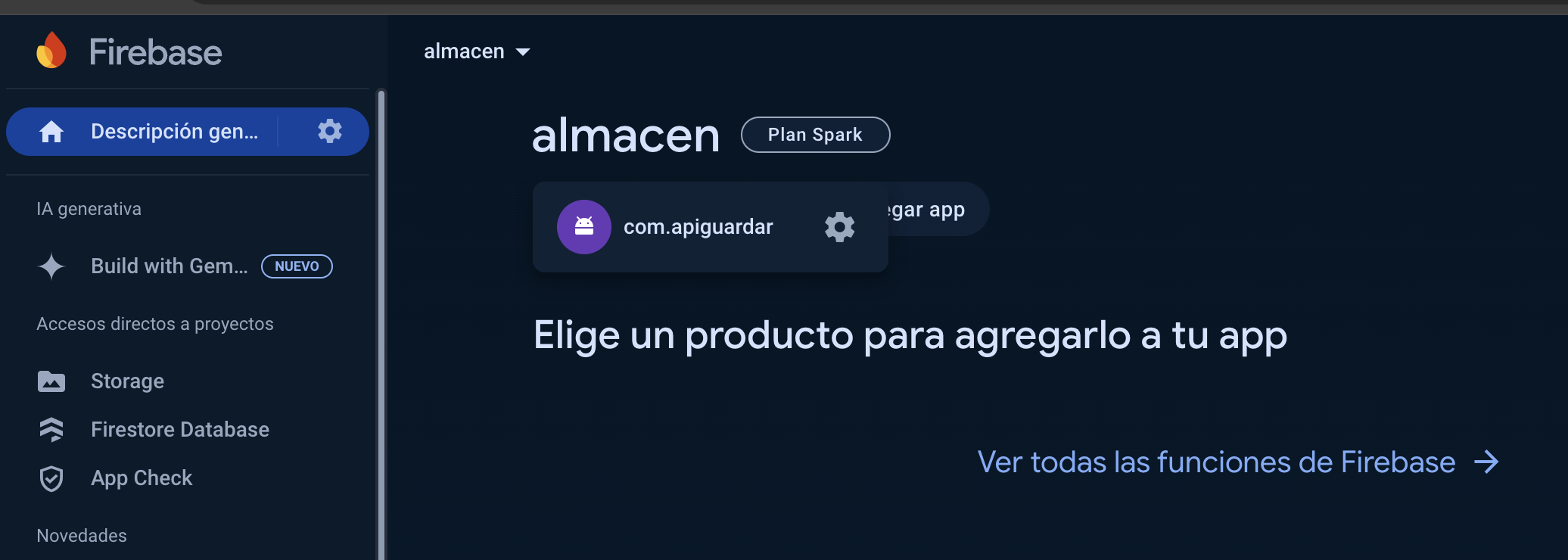
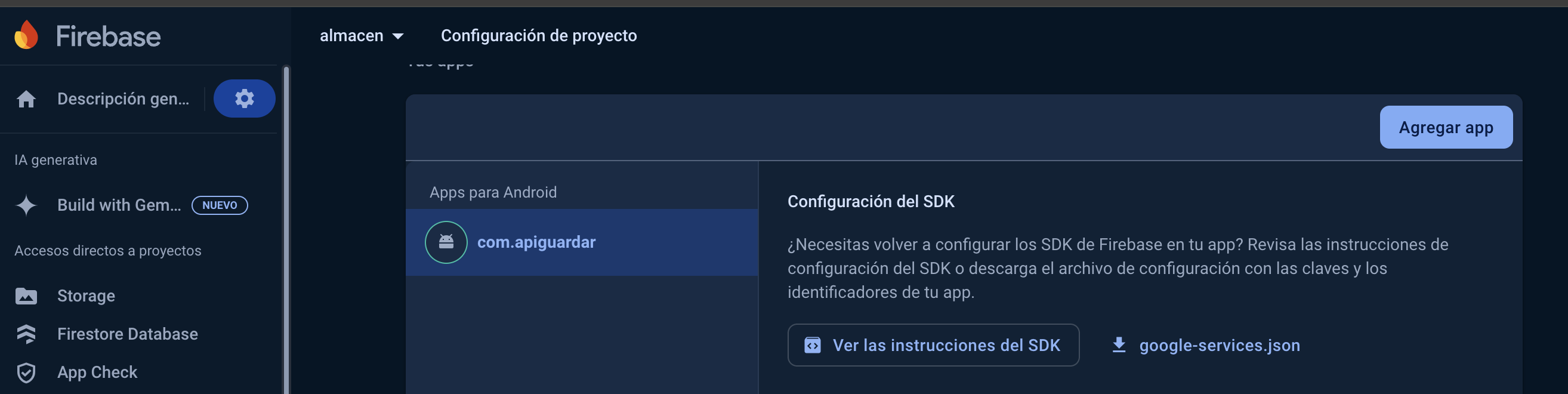
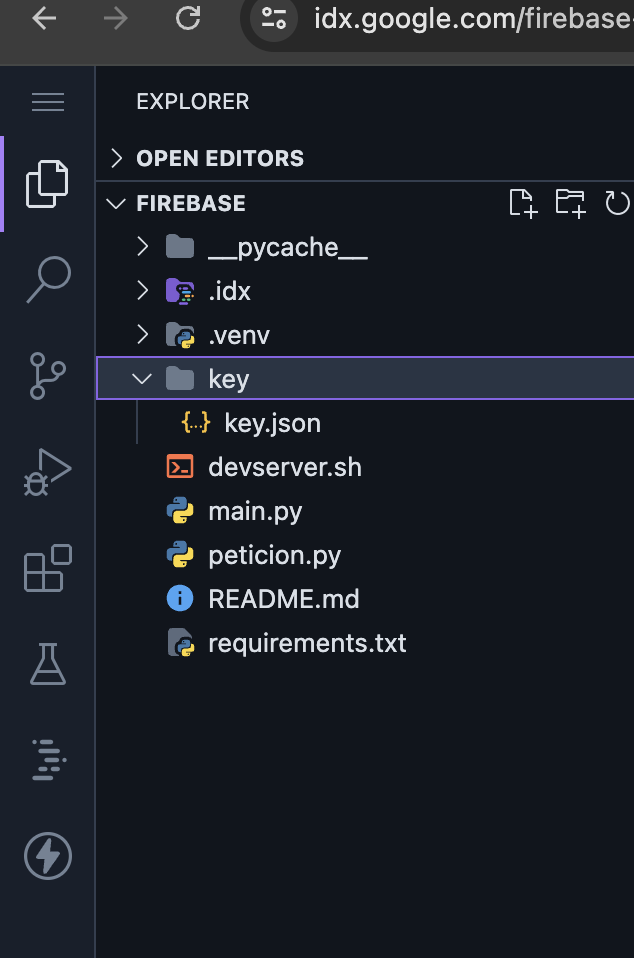
Código fuente de la aplicación que permite importar el fichero CSV
index.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
import os import csv import io import firebase_admin from firebase_admin import credentials, firestore from flask import Flask, request, render_template, jsonify # Inicializa Firebase cred = credentials.Certificate("key/key.json") firebase_admin.initialize_app(cred) # Inicializa Firestore db = firestore.client() # Configura el directorio de plantillas template_folder = os.path.join(os.path.dirname(__file__), 'templates') app = Flask(__name__, template_folder=template_folder) @app.route("/add", methods=["POST"]) def add_value(): """Recibe un valor y lo almacena en la base de datos Firestore.""" data = request.get_json() if not data or 'value' not in data: return jsonify({"error": "No se ha enviado ningún valor"}), 400 value = data['value'] try: doc_ref = db.collection('values').add({'value': value}) doc_id = doc_ref[1].id return jsonify({"id": doc_id, "value": value}), 201 except Exception as e: return jsonify({"error": str(e)}), 500 @app.route("/", methods=["GET", "POST"]) def get_values(): """Recupera todos los valores almacenados en la base de datos Firestore y renderiza la plantilla HTML.""" if request.method == "POST": file = request.files.get("file") if file and file.filename.endswith('.csv'): try: # Leer el archivo CSV stream = io.StringIO(file.stream.read().decode("UTF8"), newline=None) csv_reader = csv.DictReader(stream) # Almacenar cada fila en Firestore for row in csv_reader: db.collection('values').add(row) return jsonify({"message": "Archivo CSV procesado y datos almacenados en Firestore"}), 200 except Exception as e: return jsonify({"error": str(e)}), 500 try: docs = db.collection('values').stream() values = [{"id": doc.id, "value": doc.to_dict().get('value')} for doc in docs] return render_template('values.html', values=values) except Exception as e: return render_template('error.html', error=str(e)) if __name__ == "__main__": app.run(debug=True, host="0.0.0.0", port=int(os.environ.get("PORT", 443))) |
values.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 |
<!DOCTYPE html> <html lang="es"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Valores en Firestore</title> <style> body { background-color: #f8f9fa; } .container { margin-top: 50px; } </style> </head> <body> <div class="container"> <h1 class="text-center">Valores almacenados</h1> <!-- Formulario para agregar nuevos valores --> <form id="addValueForm" class="mb-4"> <div class="form-group"> <label for="valueInput">Nuevo valor</label> <input type="text" class="form-control" id="valueInput" placeholder="Introduce un nuevo valor" required> </div> <button type="submit" class="btn btn-primary">Agregar</button> </form> <!-- Formulario para cargar CSV --> <form id="uploadCSVForm" method="post" enctype="multipart/form-data"> <div class="form-group"> <label for="csvFile">Cargar archivo CSV</label> <input type="file" class="form-control-file" id="csvFile" name="file" accept=".csv" required> </div> <button type="submit" class="btn btn-success">Subir CSV</button> </form> <!-- Tabla de valores --> <div class="row"> <div class="col-12"> <table class="table table-striped table-bordered"> <thead class="thead-dark"> <tr> <th>ID</th> <th>Valor</th> </tr> </thead> <tbody> {% for value in values %} <tr> <td>{{ value.id }}</td> <td>{{ value.value }}</td> </tr> {% endfor %} </tbody> </table> </div> </div> </div> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/@popperjs/[email protected]/dist/umd/popper.min.js"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script> <script> // Maneja el envío del formulario de agregar valor document.getElementById('addValueForm').addEventListener('submit', function(event) { event.preventDefault(); const valueInput = document.getElementById('valueInput'); const value = valueInput.value; fetch('/add', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ value: value }) }) .then(response => response.json()) .then(data => { if (data.error) { alert('Error: ' + data.error); } else { location.reload(); } }) .catch(error => { alert('Error: ' + error); }); }); // Maneja el envío del formulario de carga de CSV document.getElementById('uploadCSVForm').addEventListener('submit', function(event) { event.preventDefault(); const formData = new FormData(this); fetch('/', { method: 'POST', body: formData }) .then(response => response.json()) .then(data => { if (data.error) { alert('Error: ' + data.error); } else { alert(data.message); location.reload(); } }) .catch(error => { alert('Error: ' + error); }); }); </script> </body> </html> |
Script en Python que permite subir un fichero CSV haciendo una petición POST
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
import requests # URL del endpoint donde se subirá el CSV url = "https://kladsfsd.vercel.app/" # Cambia esto a la URL correcta si es diferente # Ruta al archivo CSV que quieres subir csv_file_path = "fichero.csv" # Abre el archivo CSV en modo de lectura binaria with open(csv_file_path, 'rb') as csv_file: # Crea un diccionario de archivos para enviar en la solicitud files = {'file': csv_file} # Realiza la solicitud POST para subir el archivo response = requests.post(url, files=files) # Imprime la respuesta del servidor print("Status Code:", response.status_code) print("Response Text:", response.text) |
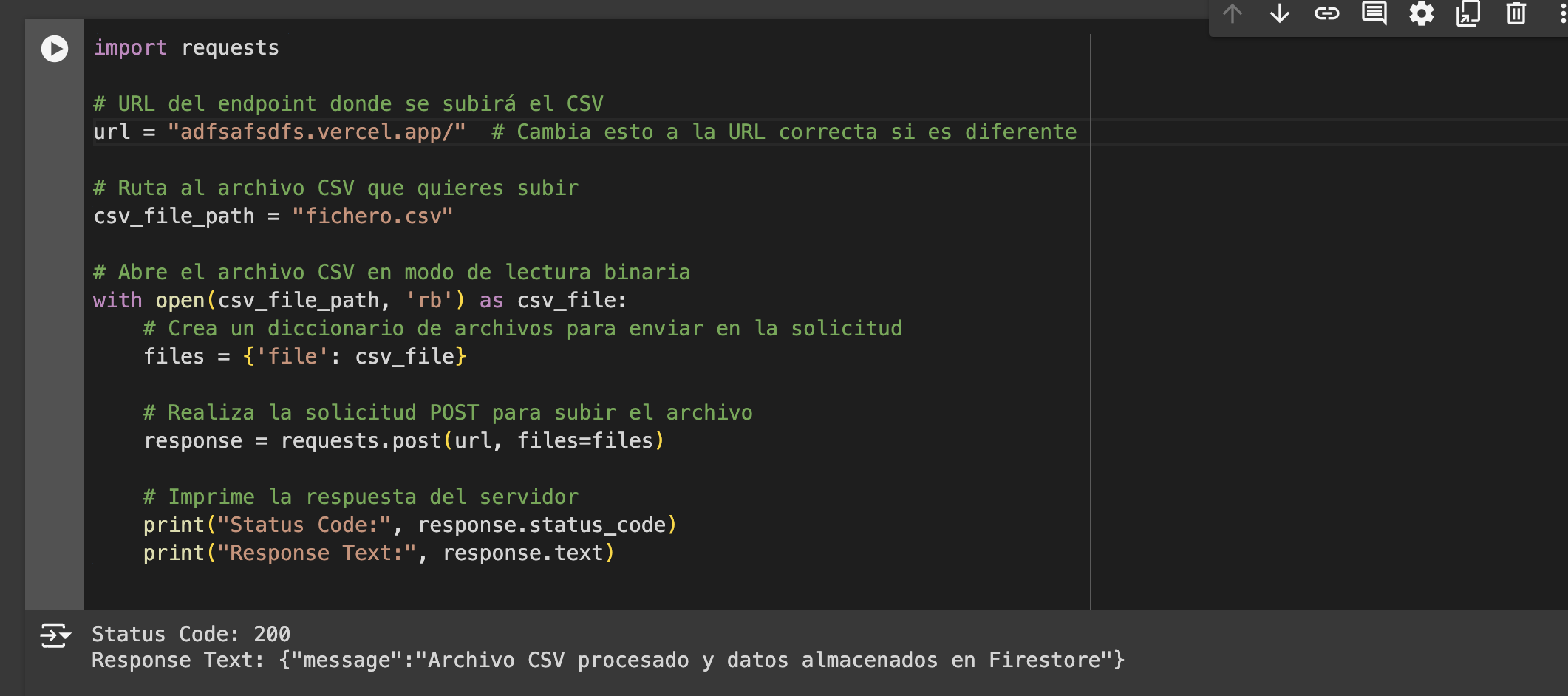