Contenidos
Explicación sobre cómo crear el API https://www.jesusninoc.com/07/30/crear-un-api-en-python-con-flask-que-inserte-y-lea-valores-en-firebase-y-desplegarlo-en-vercel/
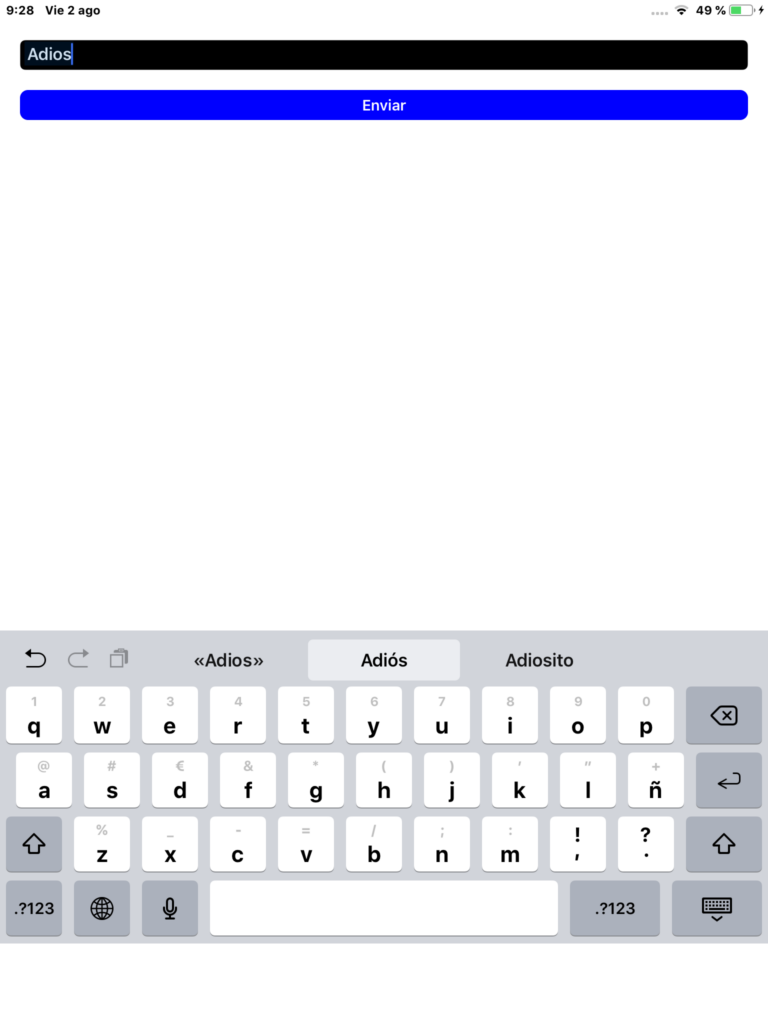
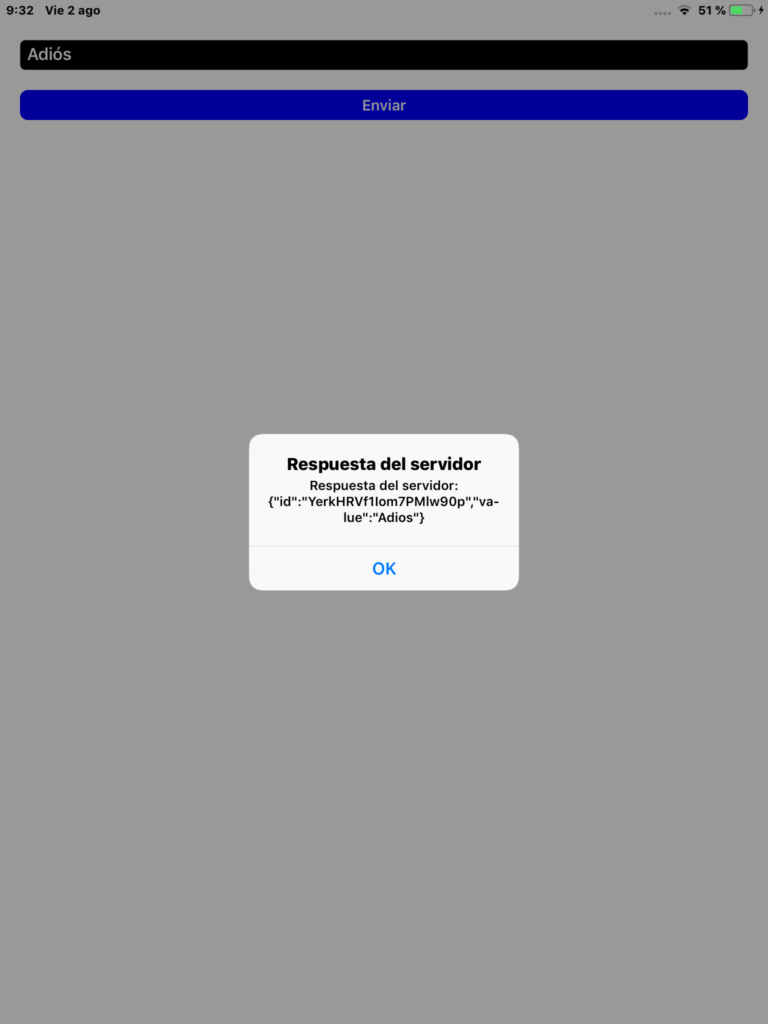

Código en Swift para iPad que inserta un valor mediante una petición POST
AppDelegate.swift
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// // AppDelegate.swift // ipad // // Created por Jesús N on 2/8/24. // import UIKit @UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate { var window: UIWindow? func application( _ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]? ) -> Bool { let window = UIWindow(frame: UIScreen.main.bounds) window.rootViewController = ViewController() window.makeKeyAndVisible() self.window = window return true } } |
ViewController.swift
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 |
// // ViewController.swift // ipad // // Created por Jesús N on 1/8/24. // import UIKit class ViewController: UIViewController { private let textField: UITextField = { let tf = UITextField() tf.placeholder = "Ingresa un valor" tf.borderStyle = .roundedRect tf.backgroundColor = .black tf.textColor = .white tf.translatesAutoresizingMaskIntoConstraints = false return tf }() private let sendButton: UIButton = { let btn = UIButton(type: .system) btn.setTitle("Enviar", for: .normal) btn.backgroundColor = .blue btn.setTitleColor(.white, for: .normal) btn.layer.cornerRadius = 8 btn.translatesAutoresizingMaskIntoConstraints = false return btn }() override func viewDidLoad() { super.viewDidLoad() setupUI() } private func setupUI() { view.backgroundColor = .white view.addSubview(textField) view.addSubview(sendButton) NSLayoutConstraint.activate([ textField.centerXAnchor.constraint(equalTo: view.centerXAnchor), textField.topAnchor.constraint(equalTo: view.safeAreaLayoutGuide.topAnchor, constant: 20), textField.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 20), textField.trailingAnchor.constraint(equalTo: view.trailingAnchor, constant: -20), sendButton.centerXAnchor.constraint(equalTo: view.centerXAnchor), sendButton.topAnchor.constraint(equalTo: textField.bottomAnchor, constant: 20), sendButton.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 20), sendButton.trailingAnchor.constraint(equalTo: view.trailingAnchor, constant: -20) ]) sendButton.addTarget(self, action: #selector(sendPostRequest), for: .touchUpInside) } @objc private func sendPostRequest() { guard let url = URL(string: "https://flask-asfasfafsd/add"), let valueToSend = textField.text else { showAlert(message: "URL inválida o campo vacío") return } let data = ["value": valueToSend] guard let jsonData = try? JSONSerialization.data(withJSONObject: data) else { showAlert(message: "Error al crear JSON") return } var request = URLRequest(url: url) request.httpMethod = "POST" request.setValue("application/json", forHTTPHeaderField: "Content-Type") request.httpBody = jsonData URLSession.shared.dataTask(with: request) { data, response, error in var resultMessage: String if let error = error { resultMessage = "Error de red: \(error.localizedDescription)" } else if let data = data { if let httpResponse = response as? HTTPURLResponse, httpResponse.statusCode == 200 { do { let responseJSON = try JSONSerialization.jsonObject(with: data) resultMessage = "Respuesta del servidor: \(responseJSON)" } catch { resultMessage = "Error al decodificar JSON: \(error.localizedDescription)" } } else { resultMessage = "Respuesta del servidor: \(String(data: data, encoding: .utf8) ?? "Desconocida")" } } else { resultMessage = "No se recibieron datos" } DispatchQueue.main.async { self.showAlert(message: resultMessage) } }.resume() } private func showAlert(message: String) { let alert = UIAlertController(title: "Respuesta del servidor", message: message, preferredStyle: .alert) alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil)) present(alert, animated: true, completion: nil) } } |