1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
import os from threading import Thread from ultralytics import YOLO def thread_safe_predict(image_path): """Predict on an image using a new YOLO model instance in a thread-safe manner and print only object names.""" print(f"Starting prediction for {image_path}") local_model = YOLO("yolov8n.pt") results = local_model.predict(image_path) # Check if results is a single object or a list if isinstance(results, list): results = results[0] # Take the first result if it's a list # Extract object names from results detected_objects = results.names # Dictionary mapping class IDs to class names # Print detected object names for box in results.boxes: for cls in box.cls: name = detected_objects[int(cls)] print(f"Detected object: {name}") def process_images(directory): """Process all .jpg images in a given directory using threads.""" # List all .jpg files in the directory image_files = [f for f in os.listdir(directory) if f.endswith('.jpg')] # Create and start a thread for each image file threads = [] for image_file in image_files: image_path = os.path.join(directory, image_file) thread = Thread(target=thread_safe_predict, args=(image_path,)) threads.append(thread) thread.start() # Join all threads for thread in threads: thread.join() # Specify the directory containing the .jpg files image_directory = './' # Process the images process_images(image_directory) |
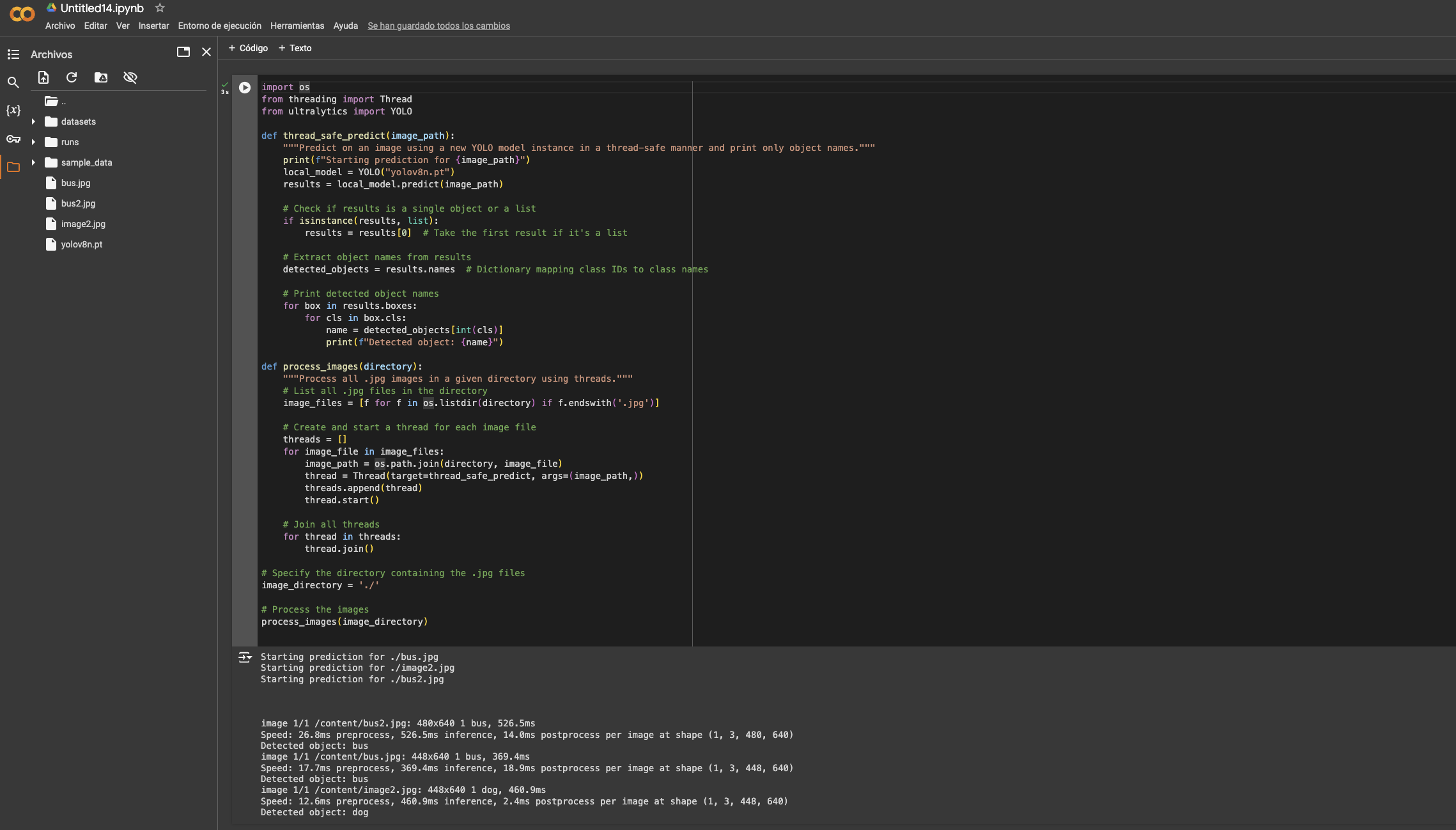