Contenidos
Cliente en iPhone que crea un archivo, lo lee y lo envía por TCP
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 |
import SwiftUI import Photos struct ContentView: View { let serverAddress = "192.168.1.55" let serverPort = 6001 var body: some View { VStack { Button("Capturar y Enviar") { captureAndSend() } .padding() .foregroundColor(.white) .background(Color.blue) .cornerRadius(8) } .padding() } func captureAndSend() { guard let window = UIApplication.shared.connectedScenes .first(where: { $0.activationState == .foregroundActive }) .flatMap({ $0 as? UIWindowScene })?.windows.first else { print("No se pudo acceder a la ventana principal") return } if let screenshot = window.captureScreen() { sendData(screenshot.pngData()) } else { print("No se pudo obtener la captura de pantalla") } } func sendData(_ data: Data?) { guard let imageData = data else { print("Datos de imagen no válidos") return } var inputStream: InputStream? var outputStream: OutputStream? Stream.getStreamsToHost(withName: serverAddress, port: serverPort, inputStream: &inputStream, outputStream: &outputStream) guard let inputStreamUnwrapped = inputStream, let outputStreamUnwrapped = outputStream else { print("Error al establecer la conexión") return } inputStreamUnwrapped.open() outputStreamUnwrapped.open() let bytesWritten = imageData.withUnsafeBytes { dataBytes in outputStreamUnwrapped.write(dataBytes.bindMemory(to: UInt8.self).baseAddress!, maxLength: imageData.count) } if bytesWritten > 0 { print("Captura de pantalla enviada con éxito") } else { print("Error al enviar la captura de pantalla") } inputStreamUnwrapped.close() outputStreamUnwrapped.close() } } extension UIView { func captureScreen() -> UIImage? { let renderer = UIGraphicsImageRenderer(bounds: bounds) return renderer.image { context in layer.render(in: context.cgContext) } } } @main struct ScreenCaptureApp: App { var body: some Scene { WindowGroup { ContentView() } } } |
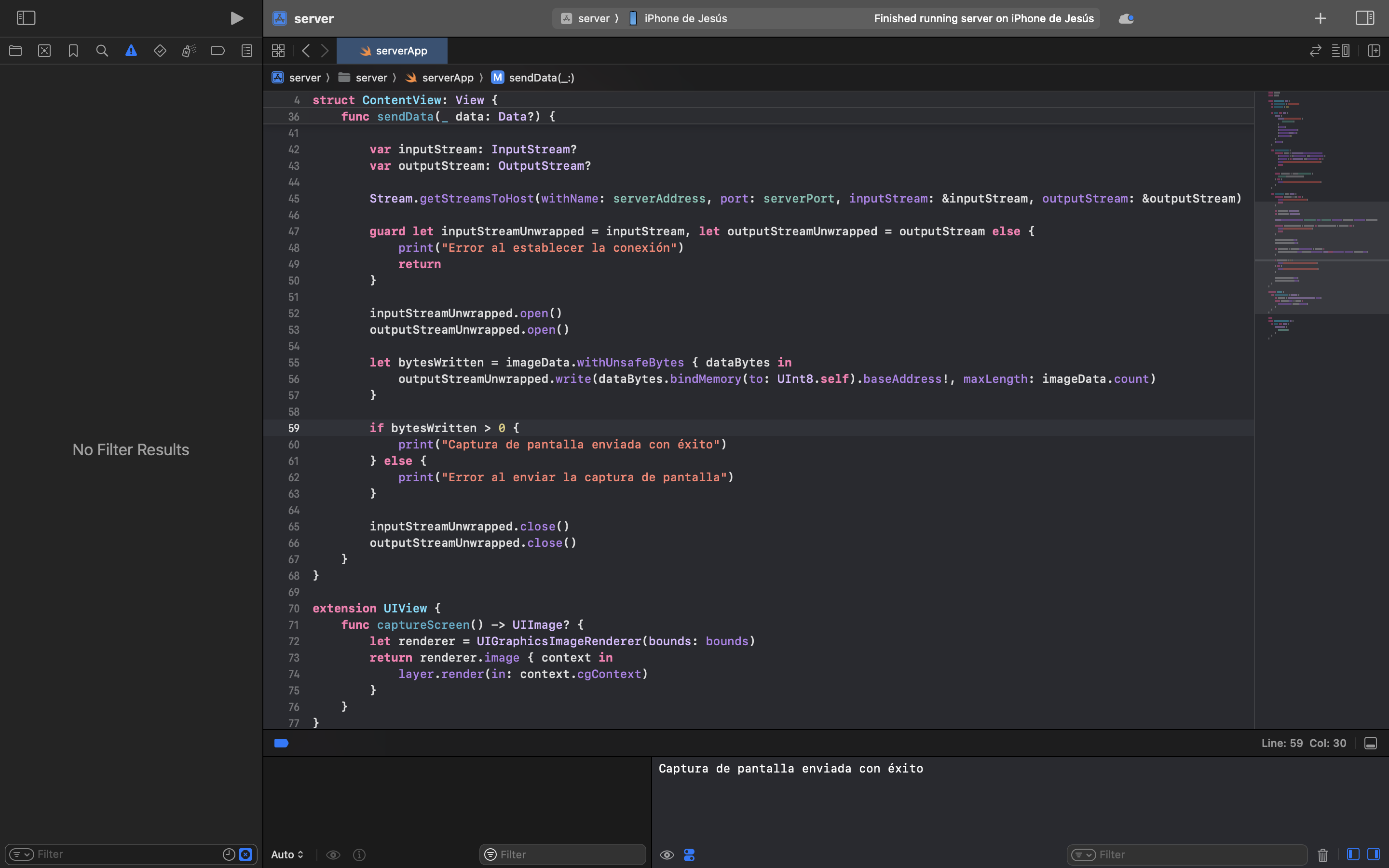
Servidor creado en IntelliJ IDEA
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import java.io.* import java.net.* fun main() { val port = 6001 try { val serverSocket = ServerSocket(port) println("Servidor TCP iniciado. Esperando conexiones...") while (true) { val clientSocket = serverSocket.accept() println("Cliente conectado desde: ${clientSocket.inetAddress.hostAddress}") val inputStream = clientSocket.getInputStream() val bufferedInputStream = BufferedInputStream(inputStream) val fileOutputStream = FileOutputStream("captura_pantalla.png") val bufferedOutputStream = BufferedOutputStream(fileOutputStream) val buffer = ByteArray(1024) var bytesRead: Int while (bufferedInputStream.read(buffer).also { bytesRead = it } != -1) { bufferedOutputStream.write(buffer, 0, bytesRead) } bufferedOutputStream.flush() println("Captura de pantalla recibida y guardada como 'captura_pantalla.png'") bufferedOutputStream.close() fileOutputStream.close() bufferedInputStream.close() inputStream.close() clientSocket.close() } } catch (e: IOException) { e.printStackTrace() } } |
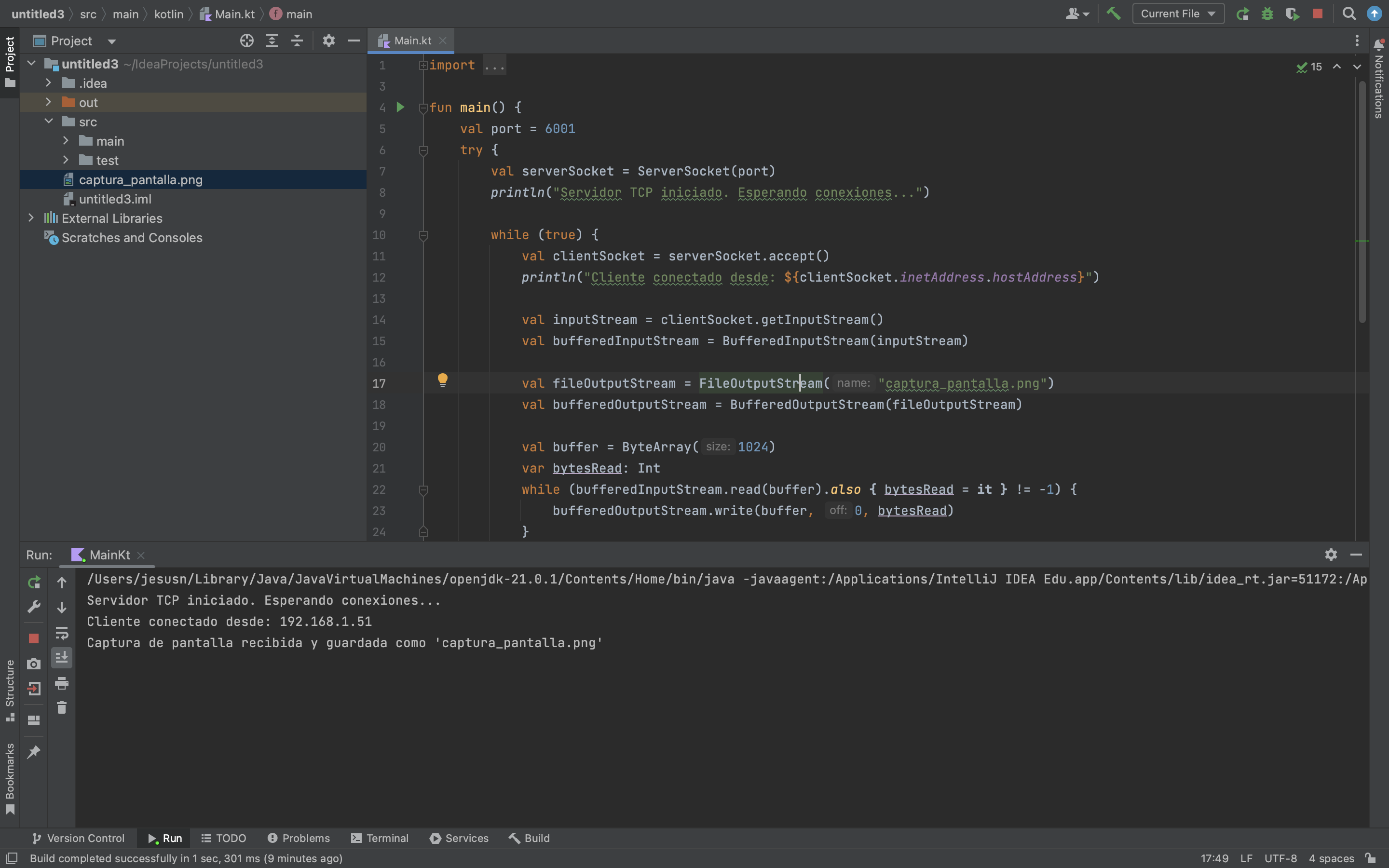