Servidor
AndroidManifest.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <application android:allowBackup="true" android:dataExtractionRules="@xml/data_extraction_rules" android:fullBackupContent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.MyApplication" tools:targetApi="31"> <activity android:name=".MainActivity" android:exported="true" android:label="@string/app_name" android:theme="@style/Theme.MyApplication"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest> |
MainActivity.kt
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
package com.example.myapplication import android.content.Context import android.os.Bundle import android.view.MotionEvent import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.layout.Box import androidx.compose.foundation.layout.fillMaxSize import androidx.compose.material3.* import androidx.compose.runtime.* import androidx.compose.ui.Alignment import androidx.compose.ui.ExperimentalComposeUiApi import androidx.compose.ui.Modifier import androidx.compose.ui.input.pointer.pointerInteropFilter import androidx.compose.ui.platform.LocalContext import com.example.myapplication.ui.theme.MyApplicationTheme import java.net.Socket class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Surface( modifier = Modifier.fillMaxSize(), color = MaterialTheme.colorScheme.background ) { val context = LocalContext.current Posicion(context) } } } } } @OptIn(ExperimentalComposeUiApi::class) @Composable fun Posicion(context: Context) { var touchPosition by remember { mutableStateOf(Pair(0f, 0f)) } var initialTouchPosition by remember { mutableStateOf(Pair(0f, 0f)) } Box( modifier = Modifier .fillMaxSize() .pointerInteropFilter { if (it.action == MotionEvent.ACTION_DOWN) { initialTouchPosition = Pair(it.x, it.y) } touchPosition = Pair(it.x, it.y) true // Indicar que el evento fue manejado }, contentAlignment = Alignment.Center ) { Text("Posición: ${touchPosition.first}, ${touchPosition.second}") Thread { val host = "192.168.1.55" // Cambia esta dirección por la del servidor val puerto = 6000 // Puerto del servidor try { val direction = getDirection(touchPosition, initialTouchPosition) direction?.let { sendDirectionToServer(it, host, puerto) } } catch (e: Exception) { e.printStackTrace() } }.start() } } fun getDirection(touchPosition: Pair<Float, Float>, initialPosition: Pair<Float, Float>): String? { val deltaX = touchPosition.first - initialPosition.first val deltaY = touchPosition.second - initialPosition.second return when { deltaX > 50 -> "derecha" deltaX < -50 -> "izquierda" deltaY > 50 -> "abajo" deltaY < -50 -> "arriba" else -> null } } fun sendDirectionToServer(direction: String, host: String, puerto: Int) { try { val cliente = Socket(host, puerto) val outputStream = cliente.getOutputStream() val mensaje = "$direction" outputStream.write(mensaje.toByteArray()) outputStream.flush() cliente.close() } catch (e: Exception) { e.printStackTrace() } } |
Cliente
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
import java.awt.MouseInfo import java.net.InetAddress import java.net.ServerSocket import java.io.BufferedReader import java.io.InputStreamReader import java.awt.Robot import java.awt.event.InputEvent fun main() { val puerto = 6000 // Puerto del servidor try { val inetAddress: InetAddress = InetAddress.getByName("192.168.1.55") val servidor = ServerSocket(puerto, 6000, inetAddress) println("Escuchando en ${servidor.localPort}") val robot = Robot() while (true) { val cliente = servidor.accept() val inputStream = cliente.getInputStream() val bufferedReader = BufferedReader(InputStreamReader(inputStream)) val mensajeRecibido = bufferedReader.readLine() println("Mensaje recibido del cliente ${cliente.inetAddress.hostAddress}:${cliente.port}: $mensajeRecibido") interpretarDireccion(mensajeRecibido, robot) bufferedReader.close() cliente.close() } // servidor.close() // No alcanzable con el ciclo while(true) } catch (e: Exception) { e.printStackTrace() } } fun interpretarDireccion(direccion: String?, robot: Robot) { direccion?.let { val currentPosition = MouseInfo.getPointerInfo().location val x = currentPosition.x val y = currentPosition.y println(it) when (it) { "izquierda" -> { robot.mouseMove(x - 10, y) // Mueve el cursor hacia la izquierda println("mover iz") } "derecha" -> { robot.mouseMove(x + 10, y) // Mueve el cursor hacia la derecha } "arriba" -> { robot.mouseMove(x, y - 10) // Mueve el cursor hacia arriba } "abajo" -> { robot.mouseMove(x, y + 10) // Mueve el cursor hacia abajo } else -> { } } } } |
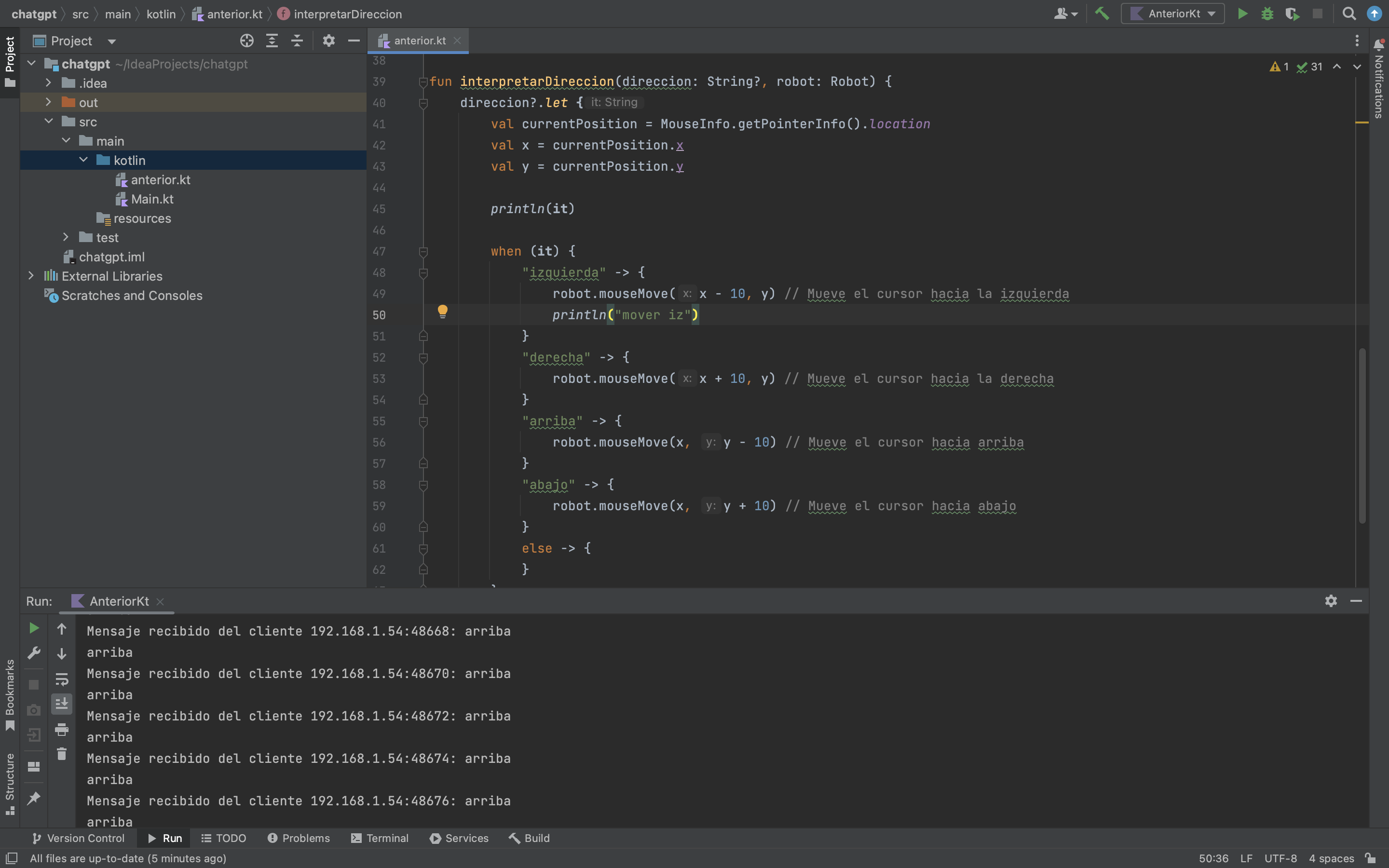